Fix "local variable referenced before assignment" in Python
Introduction
If you're a Python developer, you've probably come across a variety of errors, like the "local variable referenced before assignment" error. This error can be a bit puzzling, especially for beginners and when it involves local/global variables.
Today, we'll explain this error, understand why it occurs, and see how you can fix it.
The "local variable referenced before assignment" Error
The "local variable referenced before assignment" error in Python is a common error that occurs when a local variable is referenced before it has been assigned a value. This error is a type of UnboundLocalError
, which is raised when a local variable is referenced before it has been assigned in the local scope.
Here's a simple example:
def foo():
print(x)
x = 1
foo()
Running this code will throw the "local variable 'x' referenced before assignment" error. This is because the variable x
is referenced in the print(x)
statement before it is assigned a value in the local scope of the foo
function.
Even more confusing is when it involves global variables. For example, the following code also produces the error:
x = "Hello "
def say_hello(name):
x = x + name
print(x)
say_hello("Billy") # UnboundLocalError: local variable 'x' referenced before assignment
But wait, why does this also produce the error? Isn't x
assigned before it's used in the say_hello
function? The problem here is that x
is a global variable when assigned "Hello ". However, in the say_hello
function, it's a different local variable, which has not yet been assigned.
We'll see later in this Byte how you can fix these cases as well.
Fixing the Error: Initialization
One way to fix this error is to initialize the variable before using it. This ensures that the variable exists in the local scope before it is referenced.
Let's correct the error from our first example:
def foo():
x = 1
print(x)
foo()
In this revised code, we initialize x
with a value of 1
before printing it. Now, when you run the function, it will print 1
without any errors.
Fixing the Error: Global Keyword
Another way to fix this error, depending on your specific scenario, is by using the global
keyword. This is especially useful when you want to use a global variable inside a function.
Here's how:
x = 0
def foo():
global x
x += 1
print(x)
foo()
In this snippet, we declare x
as a global variable inside the function foo
. This tells Python to look for x
in the global scope, not the local one. Now, when you run the function, it will increment the global x
by 1
and print 1
.
Similar Error: NameError
An error that's similar to the "local variable referenced before assignment" error is the NameError
. This is raised when you try to use a variable or a function name that has not been defined yet.
Here's a simple example:
print(y)
Running this code will result in a NameError
:
$ python3 script.py
NameError: name 'y' is not defined
In this case, we're trying to print the value of y
, but y
has not been defined anywhere in the code. Hence, Python raises a NameError
. This is similar in that we are trying to use an uninitialized/undefined variable, but the main difference is that we didn't try to initialize y
anywhere else in our code.
Variable Scope in Python
Understanding the concept of variable scope can help avoid many common errors in Python, including the main error of interest in this Byte. But what exactly is variable scope?
In Python, variables have two types of scope - global and local. A variable declared inside a function is known as a local variable, while a variable declared outside a function is a global variable.
Consider this example:
x = 10 # This is a global variable
def my_function():
y = 5 # This is a local variable
print(y)
my_function()
print(x)
In this code, x
is a global variable, and y
is a local variable. x
can be accessed anywhere in the code, but y
can only be accessed within my_function
. Confusion surrounding this is one of the most common causes for the "variable referenced before assignment" error.
Conclusion
In this Byte, we've taken a look at the "local variable referenced before assignment" error and another similar error, NameError
. We also delved into the concept of variable scope in Python, which is an important concept to understand to avoid these errors. If you're seeing one of these errors, check the scope of your variables and make sure they're being assigned before they're being used.
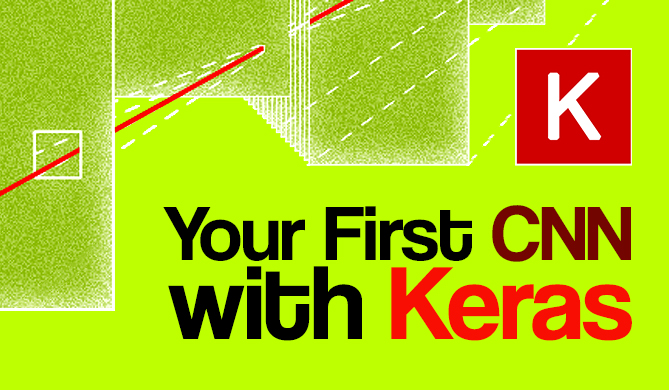
Building Your First Convolutional Neural Network With Keras
# python# artificial intelligence# machine learning# tensorflowMost resources start with pristine datasets, start at importing and finish at validation. There's much more to know. Why was a class predicted? Where was...
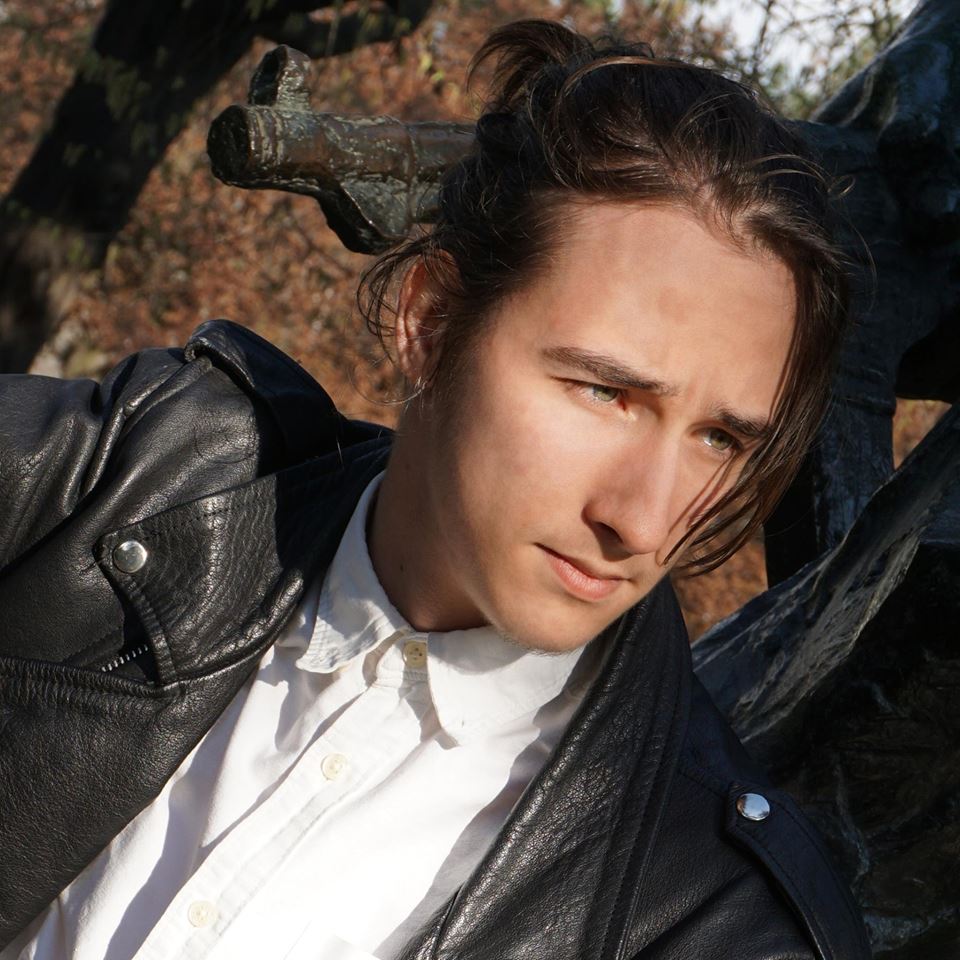