Fix the "AttributeError: module object has no attribute 'Serial'" Error in Python
Introduction
Even if you're a seasoned Python developer, you'll occasionally encounter errors that can be pretty confusing. One such error is the AttributeError: module object has no attribute 'Serial'
. This Byte will help you understand and resolve this issue.
Understanding the AttributeError
The AttributeError
in Python is raised when you try to access or call an attribute that a module, class, or instance does not have. Specifically, the error AttributeError: module object has no attribute 'Serial'
suggests that you're trying to access the Serial
attribute from a module that doesn't possess it.
For instance, if you have a module named serial
and you're trying to use the Serial
attribute from it, you might encounter this error. Here's an example:
import serial
ser = serial.Serial('/dev/ttyUSB0') # This line causes the error
In this case, the serial
module you're importing doesn't have the Serial
attribute, hence the AttributeError
.
Note: It's important to understand that Python is case-sensitive. Serial
and serial
are not the same. If the attribute exists but you're using the wrong case, you'll also encounter an AttributeError
.
Fixes for the Error
The good news is that this error is usually a pretty easy fix, even if it seems very confusing at first. Let's explore some of the solutions.
Install the Correct pyserial Module
One of the most common reasons for this error is the incorrect installation of the pyserial
module. The Serial
attribute is a part of the pyserial
module, which is used for serial communication in Python.
You might have installed a module named serial
instead of pyserial
(this is more common than you think!). To fix this, you need to uninstall the incorrect module and install the correct one.
$ pip uninstall serial
$ pip install pyserial
After running these commands, your issue may be resolved. Now you can import Serial
from pyserial
and use it in your code:
from pyserial import Serial
ser = Serial('/dev/ttyUSB0') # This line no longer causes an error
If this didn't fix the error, keep reading.
Rename your serial.py File
For how much Python I've written in my career, you'd think that I wouldn't make this simple mistake as much as I do...
Another possibility is that the Python interpreter gets confused when there's a file in your project directory with the same name as a module you're trying to import. This is another common source of the AttributeError
error.
Let's say, for instance, you have a file named serial.py
in your project directory (or maybe your script itself is named serial.py
). When you try to import serial
, Python might import your serial.py
file instead of the pyserial
module, leading to the AttributeError
.
The solution here is simple - rename your serial.py
file to something else.
$ mv serial.py my_serial.py
Conclusion
In this Byte, we've explored two common causes of the AttributeError: module object has no attribute 'Serial'
error in Python: installing the wrong pyserial
module, and having a file in your project directory that shares a name with a module you're trying to import. By installing the correct module or renaming conflicting files, you should be able to eliminate this error.
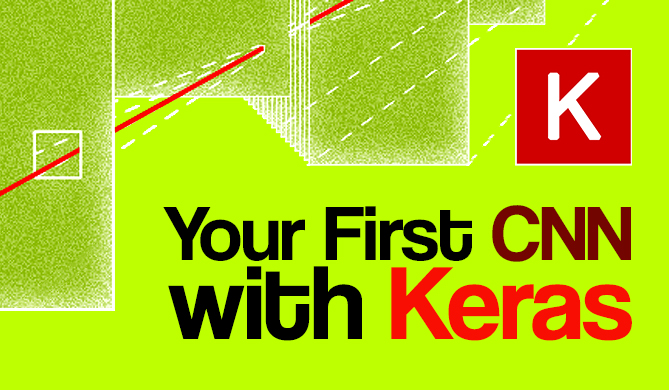
Building Your First Convolutional Neural Network With Keras
# python# artificial intelligence# machine learning# tensorflowMost resources start with pristine datasets, start at importing and finish at validation. There's much more to know. Why was a class predicted? Where was...
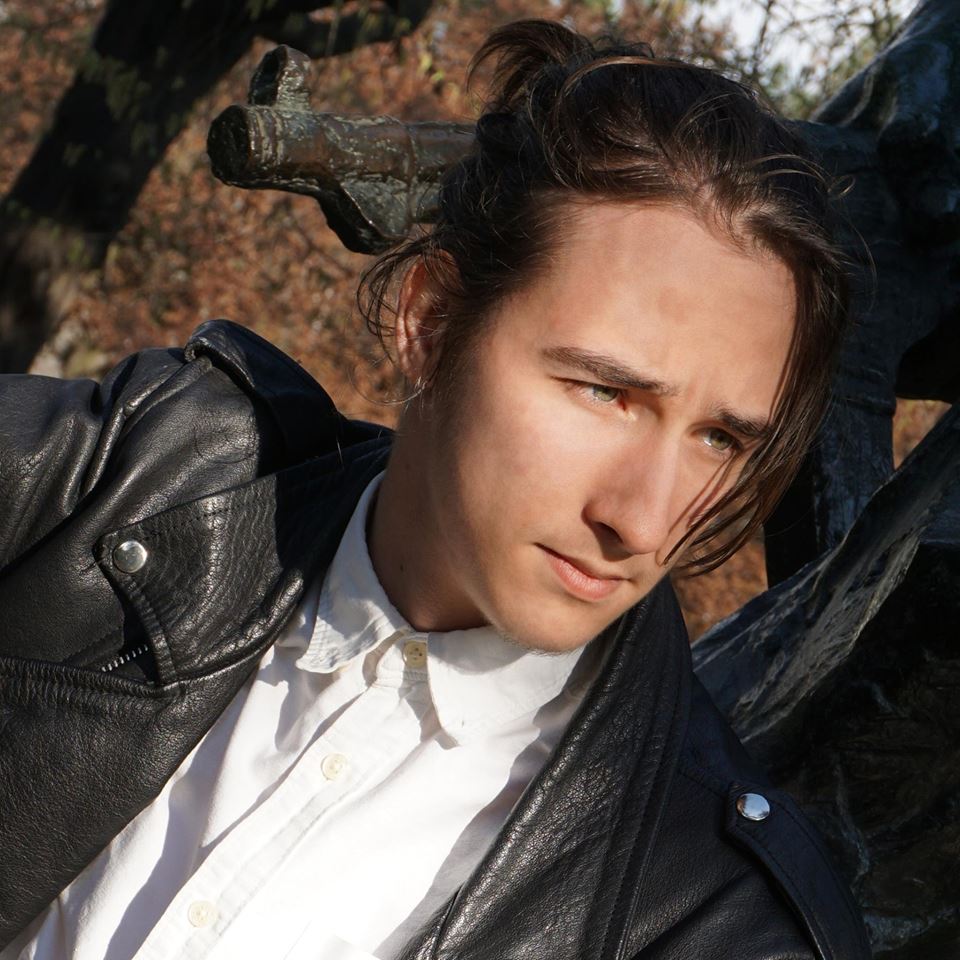