How to Pass Multiple Arguments to the map() Function in Python
Introduction
The goal of Python, with its rich set of built-in functions, is to allow developers to accomplish complex tasks with relative ease. One such powerful, yet often overlooked, function is the map()
function. The map()
function will execute a given function over a set of items, but how do we pass additional arguments to the provided function?
In this Byte, we'll be exploring the map()
function and how to effectively pass multiple arguments to it.
The map() Function in Python
The map()
function in Python is a built-in function that applies a given function to every item of an iterable (like list, tuple etc.) and returns a list of the results.
def square(number):
return number ** 2
numbers = [1, 2, 3, 4, 5]
squared = map(square, numbers)
print(list(squared)) # Output: [1, 4, 9, 16, 25]
In this snippet, we've defined a function square()
that takes a number and returns its square. We then use the map()
function to apply this square()
function to each item in the numbers
list.
Why Pass Multiple Arguments to map()?
You might be wondering, "Why would I need to pass multiple arguments to map()
?" Well, there are scenarios where you might have a function that takes more than one argument, and you want to apply this function to multiple sets of data simultaneously.
Not every function we provide to map()
will take only one argument. What if, instead of a squared
function, we have a more generic math.pow
function, and one of the arguments is what number to raise the item to. How do we handle a case like this?
Or maybe you have two lists of numbers, and you want to find the product of corresponding numbers from these lists. This is another case where passing multiple arguments to map()
can come be helpful.
How to Pass Multiple Arguments to map()
There are a few different types of cases in which you'd want to pass multiple arguments to map()
, two of which we mentioned above. We'll walk through both of those cases here.
Multiple Iterables
Passing multiple arguments to the map()
function is simple once you understand how to do it. You simply pass additional iterables after the function argument, and map()
will take items from each iterable and pass them as separate arguments to the function.
Here's an example:
def multiply(x, y):
return x * y
numbers1 = [1, 2, 3, 4, 5]
numbers2 = [6, 7, 8, 9, 10]
result = map(multiply, numbers1, numbers2)
print(list(result)) # Output: [6, 14, 24, 36, 50]
Note: Make sure that the number of arguments in the function should match the number of iterables passed to map()
!
In the example above, we've defined a function multiply()
that takes two arguments and returns their product. We then pass this function, along with two lists, to the map()
function. The map()
function applies multiply()
to each pair of corresponding items from the two lists, and returns a new list with the results.
Multiple Arguments, One Iterable
Continuing with our math.pow
example, let's see how we can still use map()
to run this function on all items of an array.
The first, and probably simplest, way is to not use map()
at all, but to use something like list comprehension instead.
import math
numbers = [1, 2, 3, 4, 5]
res = [math.pow(n, 3) for n in numbers]
print(res) # Output: [1.0, 8.0, 27.0, 64.0, 125.0]
This is essentiall all map()
really is, but it's not as compact and neat as using a convenient function like map()
.
Now, let's see how we can actually use map()
with a function that requires multiple arguments:
import math
import itertools
numbers = [1, 2, 3, 4, 5]
res = map(math.pow, numbers, itertools.repeat(3, len(numbers)))
print(list(res)) # Output: [1.0, 8.0, 27.0, 64.0, 125.0]
This may seem a bit more complicated at first, but it's actually very simple. We use a helper function, itertools.repeat
, to create a list the same length as numbers
and with only values of 3
.
So the output of itertools.repeat(3, len(numbers))
, when converted to a list, is just [3, 3, 3, 3, 3]
. This works because we're now passing two lists of the same length to map()
, which it happily accepts.
Conclusion
The map()
function is particularly useful when working with multiple iterables, as it can apply a function to the elements of these iterables in pairs, triples, or more. In this Byte, we've covered how to pass multiple arguments to the map()
function and how to work with multiple iterables.
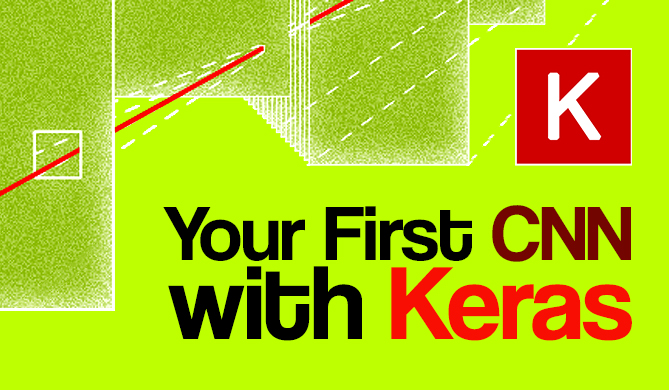
Building Your First Convolutional Neural Network With Keras
# python# artificial intelligence# machine learning# tensorflowMost resources start with pristine datasets, start at importing and finish at validation. There's much more to know. Why was a class predicted? Where was...
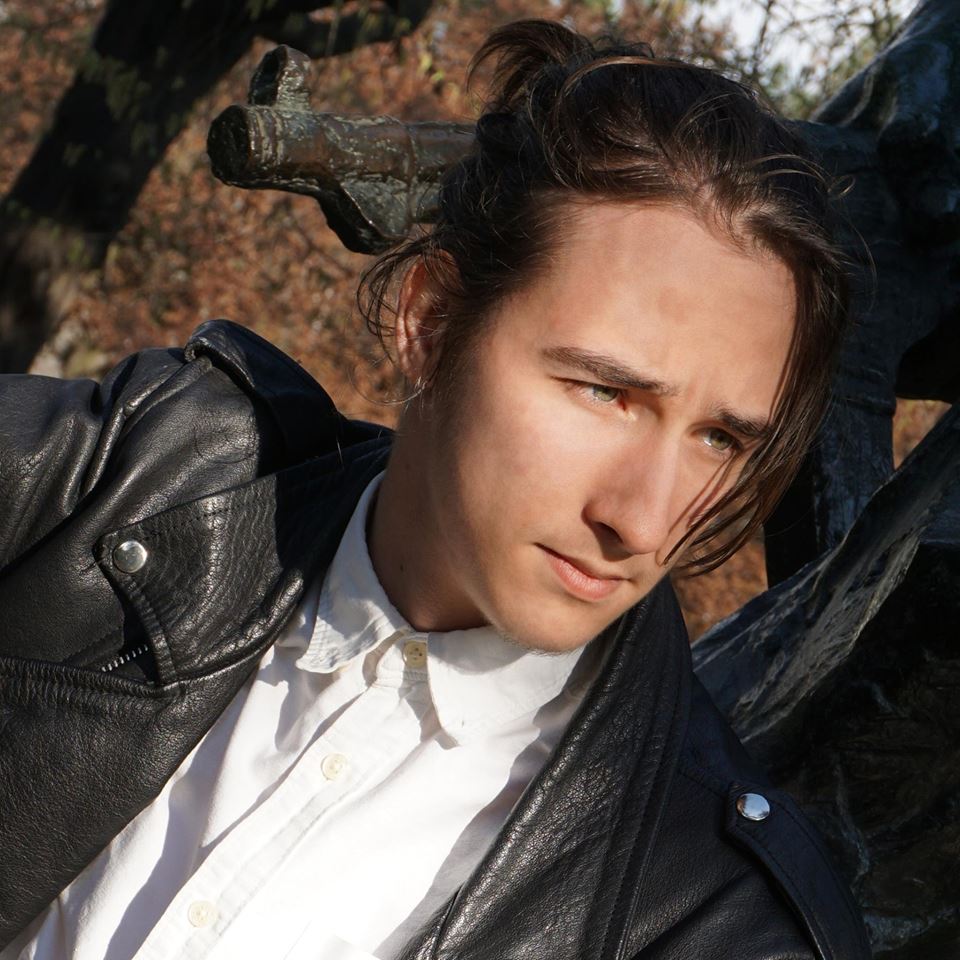