Using cla(), clf(), and close() to Clear a Plot in Matplotlib
Introduction
In Matplotlib, there are a number of ways to close plots, including the functions cla()
, clf()
, and close()
. These functions are helpful tools for managing our plots. But when should we use each one? Let's take a look.
Using cla() to Clear a Plot
The cla()
function stands for "clear axis". It is used to clear the current axis in a figure, basically removing all plotted data, labels, titles, or other elements from the axis, while leaving the figure itself intact. It's like erasing a drawing but leaving the canvas untouched. Here's a simple example:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4])
plt.ylabel('Numbers')
plt.cla()
plt.show()
After calling plt.cla()
, the plot is cleared, but the figure is still open. You can add new plots to the same figure without having to create a new one. Here's what that would look like with the previous example:
Note: cla()
only clears the current axis. If you have multiple axes in your figure, you'll need to select each one and call cla()
to clear them all.
Using clf() to Clear a Figure
clf()
, or "clear figure", is a step up from cla()
. Instead of just clearing the axis, clf()
clears the entire figure. This includes all axes, titles, labels, and plotted data. It's like throwing away the canvas and getting a brand new one. Let's see it how it works:
import matplotlib.pyplot as plt
plt.figure(1) # first figure
plt.subplot(211) # the first subplot in the first figure
plt.plot([1, 2, 3])
plt.subplot(212) # the second subplot in the first figure
plt.plot([4, 5, 6])
plt.clf()
plt.show()
After calling plt.clf()
, the figure and all their subplots are cleared. You can then start fresh with a new plot.
Using close() to Close a Window or Figure
Finally, we have close()
. This function closes the window, along with any figures and plots it contains. It's like not just throwing away the canvas, but also leaving the studio. Here's how you use it:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4])
plt.ylabel('Numbers')
plt.show(block=False)
plt.pause(3)
plt.close()
After calling plt.close()
, the figure window will close completely after 3 seconds. Any attempt to plot to it will result in an error, unless you create a new figure.
Note: The trick here is to use the param block=False
so that the code can continue to execute past plt.show()
and eventually close using the plt.close()
method. If you don't set the block
param, the execution will stop there and not reach the close()
method.
Differences Between cla(), clf(), and close()
In the previous sections, we've looked at how cla()
, clf()
, and close()
can be used to manage our plots and figures in Matplotlib. However, it's important to understand the differences between these three functions to use them in the right circumstances.
cla()
or clear axis
essentially clears an axis, leaving an empty plot or subplot behind, but keeping the axis in tact. It's useful when you need to plot new data on the same axis. Here's an example:
clf()
or clear figure
clears the entire figure including all its axes, but keeps the window opened, as if it was just created. So, if you're planning to create a completely new plot, clf()
is the way to go.
Finally, close()
closes a window completely, which will be helpful when you're done with your plot and want to free up the memory.
While cla()
and clf()
are used inside a plotting script, close()
is generally used at the end of the script. The cla()
and clf()
methods are often used when you're showing an interactive plot or one that changes over time.
Conclusion
In this Byte, we've explored the differences between cla()
, clf()
, and close()
in Matplotlib. These functions provide us with different levels of control over how we clear our plots, figures, and windows. Understanding when and how to use these commands can help you manage your plots better.
Remember, cla()
clears an axis, clf()
clears an entire figure, and close()
closes a window completely. So, whether you're updating a single plot, creating a new figure, or wrapping up your work, Matplotlib likely has a method for your use-case.
You might also like...
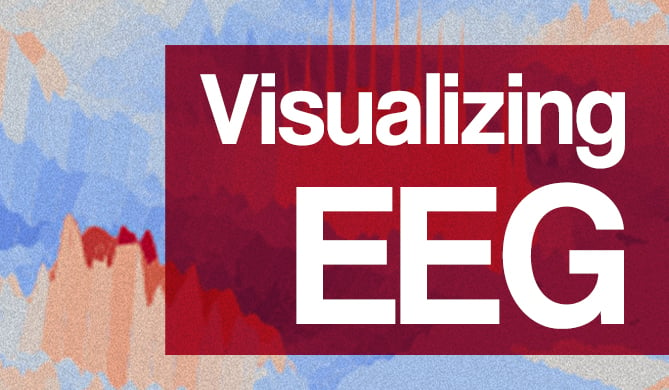
Data Visualization in Python: Visualizing EEG Brainwave Data
# python# matplotlib# seaborn# data visualizationElectroencephalography (EEG) is the process of recording an individual's brain activity - from a macroscopic scale. It's a non-invasive (external) procedure and collects aggregate, not...
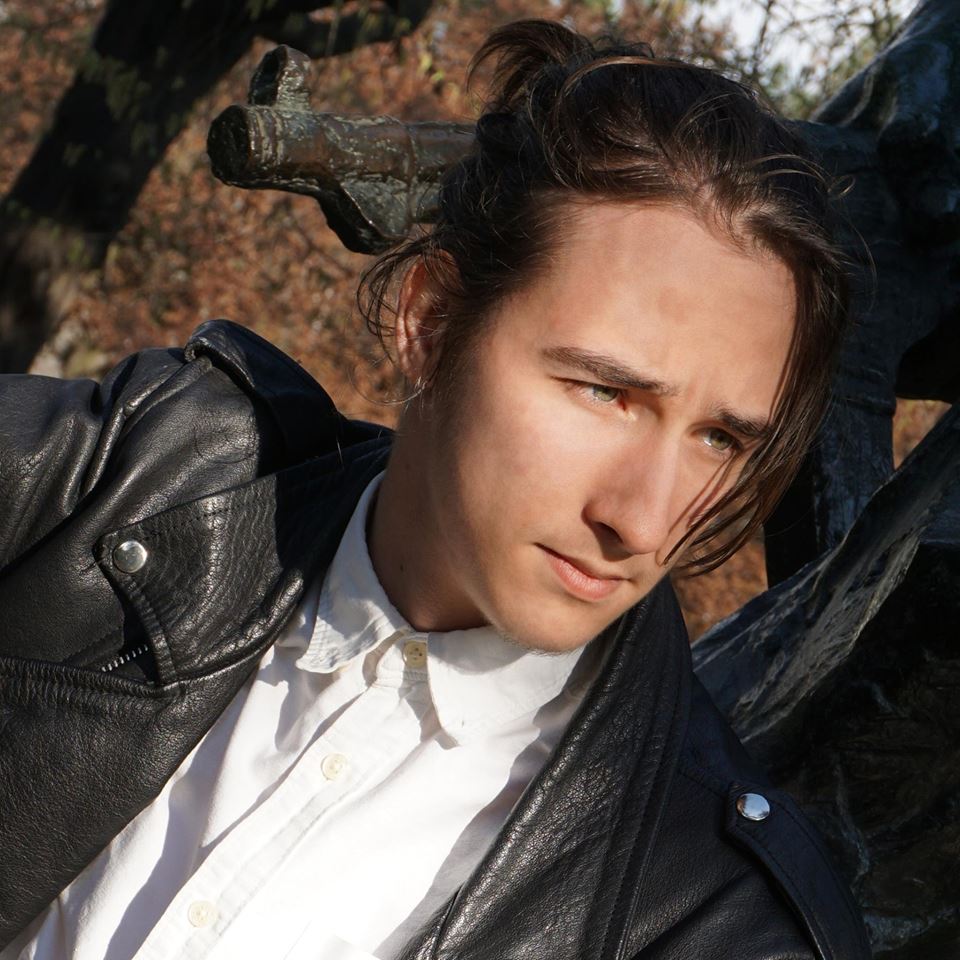
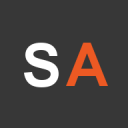